- FREE Express Shipping On Orders $99+
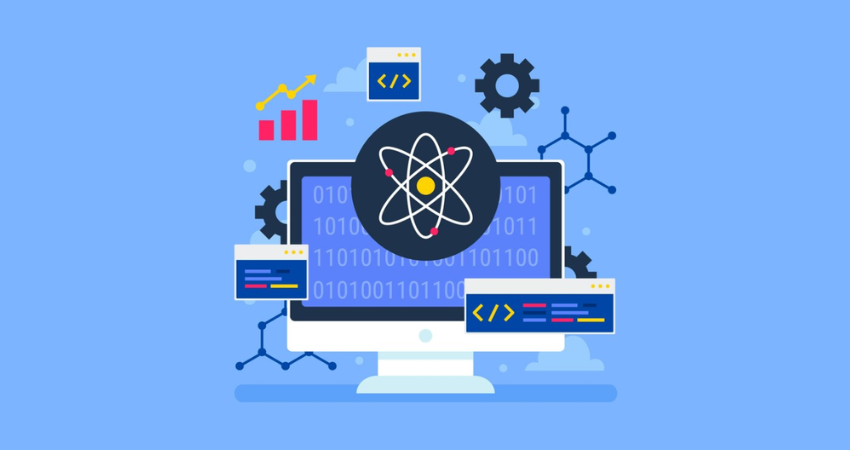
Mastering MERN: A Step-by-Step Guide to Building Your First Web App
In the rapidly evolving landscape of web development, leveraging the right technology stack can make or break your project. Among the various stacks available, MERN stands out as a powerful combination for building modern, scalable web applications. Comprising MongoDB, Express.js, React, and Node.js, the MERN stack offers a comprehensive solution for both client-side and server-side development. For businesses seeking robust software development services, understanding and mastering MERN can be a game-changer. This guide provides a step-by-step approach to building your first web app with MERN, aimed at software development companies and professionals in the field.
Understanding the MERN Stack
Before diving into the development process, it’s crucial to understand the components that make up the MERN stack:
- MongoDB: A NoSQL database that uses a flexible, JSON-like document structure, making it ideal for handling large volumes of data.
- Express.js: A web application framework for Node.js, providing a robust set of features for web and mobile applications.
- React: A JavaScript library for building user interfaces, particularly single-page applications where you can build complex UIs from small and isolated pieces of code called “components.”
- Node.js: A JavaScript runtime built on Chrome’s V8 JavaScript engine, enabling server-side scripting and building scalable network applications.
Step 1: Setting Up Your Development Environment
To start with MERN, ensure you have the following installed:
- Node.js and npm: Download and install Node.js from the official Node.js website. npm (Node Package Manager) comes bundled with Node.js.
- MongoDB: Install MongoDB from the MongoDB website. Alternatively, you can use MongoDB Atlas, a cloud database service.
- Code Editor: Use a code editor like Visual Studio Code (VS Code) for an optimal development experience.
Step 2: Initializing Your Project
Create a new directory for your project and navigate into it using your terminal. Then, initialize a new Node.js project:
mkdir mern-app
cd mern-app
npm init -y
This command creates a package.json file, which keeps track of your project’s dependencies and scripts.
Step 3: Setting Up Express.js and Node.js
Install Express.js and other necessary middleware:
npm install express body-parser mongoose cors
Create a server.js file to set up your Express server:
const express = require(‘express’);
const bodyParser = require(‘body-parser’);
const mongoose = require(‘mongoose’);
const cors = require(‘cors’);
const app = express();
const port = process.env.PORT || 5000;
// Middleware
app.use(bodyParser.json());
app.use(cors());
// MongoDB connection
mongoose.connect(‘mongodb://localhost:27017/mern-app’, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log(‘MongoDB connected’))
.catch(err => console.log(err));
// Routes
app.get(‘/’, (req, res) => {
res.send(‘Hello World!’);
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
Step 4: Setting Up MongoDB with Mongoose
Mongoose is an ODM (Object Data Modeling) library for MongoDB and Node.js. It manages relationships between data, provides schema validation, and is used to translate between objects in code and the representation of those objects in MongoDB.
Create a models directory and add a file named Item.js:
const mongoose = require(‘mongoose’);
const Schema = mongoose.Schema;
const ItemSchema = new Schema({
name: {
type: String,
required: true
},
date: {
type: Date,
default: Date.now
}
});
module.exports = mongoose.model(‘item’, ItemSchema);
Step 5: Creating API Routes
Create a routes directory and add a file named items.js:
const express = require(‘express’);
const router = express.Router();
// Item Model
const Item = require(‘../models/Item’);
// @route GET api/items
// @desc Get All Items
// @access Public
router.get(‘/’, (req, res) => {
Item.find()
.sort({ date: -1 })
.then(items => res.json(items));
});
// @route POST api/items
// @desc Create An Item
// @access Public
router.post(‘/’, (req, res) => {
const newItem = new Item({
name: req.body.name
});
newItem.save().then(item => res.json(item));
});
// @route DELETE api/items/:id
// @desc Delete An Item
// @access Public
router.delete(‘/:id’, (req, res) => {
Item.findById(req.params.id)
.then(item => item.remove().then(() => res.json({ success: true })))
.catch(err => res.status(404).json({ success: false }));
});
module.exports = router;
In server.js, use these routes:
const items = require(‘./routes/items’);
app.use(‘/api/items’, items);
Step 6: Setting Up React
Navigate to your project directory and create a React application using Create React App:
npx create-react-app client
Once the setup is complete, navigate into the client directory:
cd client
Step 7: Connecting React to Express
In the client directory, install Axios for making HTTP requests:
npm install axios
Modify the package.json file in the client directory to add a proxy for the backend:
“proxy”: “http://localhost:5000”
Step 8: Building the Frontend with React
Create a components directory in src and add a file named ItemList.js:
import React, { Component } from ‘react’;
import axios from ‘axios’;
class ItemList extends Component {
state = {
items: []
};
componentDidMount() {
axios.get(‘/api/items’)
.then(res => {
this.setState({ items: res.data });
})
.catch(err => {
console.log(err);
});
}
render() {
return (
<div>
<h1>Items</h1>
<ul>
{this.state.items.map(item => (
<li key={item._id}>{item.name}</li>
))}
</ul>
</div>
);
}
}
export default ItemList;
Modify App.js to include the ItemList component:
import React from ‘react’;
import ‘./App.css’;
import ItemList from ‘./components/ItemList’;
function App() {
return (
<div className=”App”>
<header className=”App-header”>
<h1>My MERN App</h1>
<ItemList />
</header>
</div>
);
}
export default App;
Step 9: Running the Application
In the mern-app directory, open two terminals:
In the first terminal, start the Express server:
node server.js
In the second terminal, navigate to the client directory and start the React development server:
npm start
Conclusion
Congratulations! You have successfully built a simple web application using the MERN stack. This project serves as a solid foundation upon which you can build more complex applications. For software development services and companies, mastering the MERN stack opens up new opportunities for creating high-performance, scalable web applications. The knowledge gained from this guide will be invaluable in delivering top-notch software solutions to clients, ensuring your company stays at the forefront of technological innovation.
By following these steps, you have not only built a functional web app but also gained insight into the seamless integration of MongoDB, Express.js, React, and Node.js. This understanding will be instrumental in addressing client needs and delivering comprehensive software development services that stand out in the competitive market. Whether you are an individual developer or part of a custom software development company, the MERN stack offers a powerful toolkit for building the future of web applications
To read more about The Future of Document Security: ECM Software
.