- FREE Express Shipping On Orders $99+
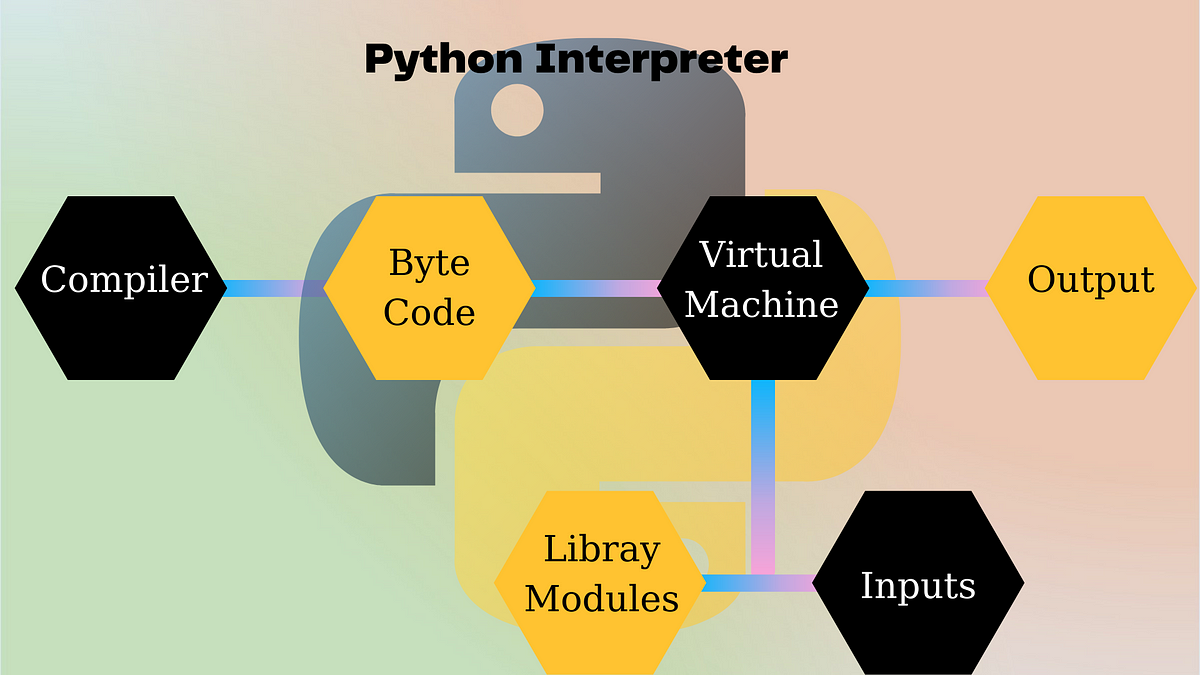
Building a React Navigation Interpreter in Python: A Comprehensive Guide
Navigating through the intricacies of web development often requires the integration of various technologies. One such powerful combination is using React Navigation for handling navigation in a React application and creating an interpreter in Python to streamline and automate certain tasks. This comprehensive guide will walk you through the process of building a React Navigation interpreter in Python, ensuring you can leverage the strengths of both technologies effectively.
Introduction to React Navigation and Python Interpreters
React Navigation is a popular library for routing and navigation in React Native applications. It allows developers to create seamless navigation experiences with minimal effort. On the other hand, Python interpreters are programs that read and execute Python code, making it possible to automate tasks, process data, and interact with various APIs.
Prerequisites
Before diving into the implementation, ensure you have the following:
- Basic understanding of React and React Navigation.
- Familiarity with Python programming.
- Installed Node.js and npm.
- Installed Python on your system.
Setting Up the React Project
Initialize a React Native Project:
bash
Copy code
npx react-native init NavigationInterpreterApp
cd NavigationInterpreterApp
- Install React Navigation:
bash
Copy code
npm install @react-navigation/native
npm install @react-navigation/stack
npm install react-native-screens react-native-safe-area-context
- Set Up React Navigation:
javascript
Copy code
// In your App.js
import ‘react-native-gesture-handler’;
import * as React from ‘react’;
import { NavigationContainer } from ‘@react-navigation/native’;
import { createStackNavigator } from ‘@react-navigation/stack’;
import HomeScreen from ‘./screens/HomeScreen’;
import DetailsScreen from ‘./screens/DetailsScreen’;
const Stack = createStackNavigator();
function App() {
return (
<NavigationContainer>
<Stack.Navigator initialRouteName=”Home”>
<Stack.Screen name=”Home” component={HomeScreen} />
<Stack.Screen name=”Details” component={DetailsScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
export default App;
- Building the Python Interpreter
Install Required Python Packages:
bash
Copy code
pip install requests
- Create the Python Script:
python
Copy code
import requests
class NavigationInterpreter:
def __init__(self, base_url):
self.base_url = base_url
def navigate(self, route, params=None):
response = requests.post(f'{self.base_url}/navigate’, json={‘route’: route, ‘params’: params})
return response.json()
# Example usage
if __name__ == “__main__”:
interpreter = NavigationInterpreter(‘http://localhost:5000’)
result = interpreter.navigate(‘Details’, {‘itemId’: 42})
print(result)
- Integrating the Python Interpreter with React Navigation
To bridge the gap between React Navigation and the Python interpreter, you can set up a simple Flask server to handle navigation requests.
Install Flask:
bash
Copy code
pip install Flask
- Create the Flask Server:
python
Copy code
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route(‘/navigate’, methods=[‘POST’])
def navigate():
data = request.json
route = data.get(‘route’)
params = data.get(‘params’)
# Logic to handle navigation
return jsonify({‘status’: ‘success’, ‘route’: route, ‘params’: params})
if __name__ == “__main__”:
app.run(debug=True)
- Connect Flask Server to React App: Modify the Python script to send navigation requests to the Flask server, which will then handle navigation within the React Native application.
Conclusion
By following this comprehensive guide, you have learned how to build a React Navigation interpreter in Python. This powerful combination allows for seamless integration and automation, enhancing the capabilities of your web development projects. Whether you’re handling complex navigational structures or automating repetitive tasks, leveraging React Navigation and Python interpreters can significantly streamline your workflow.